register_graphql_mutation
Given a Mutation Name and Config array, this adds a Mutation to the Schema
register_graphql_mutation( string $mutation_name, array $config );
Parameters
- $mutation_name (string): The name of the mutation
- $config (array): Configuration for the mutation
- $description (string): Description of the mutation type.
- $inputFields (array): The input fields for the mutation
- $type (string): The name of the Type in the Schema the field should accept. Note: ObjectTypes cannot be used as Input Types.
- $description (string): The field description.
- $outputFields (array): The output field for the mutation (what can be asked for in response)
- $mutateAndGetPayload (function): How the mutation should resolve
Source
File: access-functions.php
Example
Here is a basic example of registering a mutation:
# This function registers a mutation to the Schema.
# The first argument, in this case `exampleMutation`, is the name of the mutation in the Schema
# The second argument is an array to configure the mutation.
# The config array accepts 3 key/value pairs for: inputFields, outputFields and mutateAndGetPayload.
register_graphql_mutation( 'exampleMutation', [
# inputFields expects an array of Fields to be used for inputting values to the mutation
'inputFields' => [
'exampleInput' => [
'type' => 'String',
'description' => __( 'Description of the input field', 'your-textdomain' ),
]
],
# outputFields expects an array of fields that can be asked for in response to the mutation
# the resolve function is optional, but can be useful if the mutateAndPayload doesn't return an array
# with the same key(s) as the outputFields
'outputFields' => [
'exampleOutput' => [
'type' => 'String',
'description' => __( 'Description of the output field', 'your-textdomain' ),
'resolve' => function( $payload, $args, $context, $info ) {
return isset( $payload['exampleOutput'] ) ? $payload['exampleOutput'] : null;
}
]
],
# mutateAndGetPayload expects a function, and the function gets passed the $input, $context, and $info
# the function should return enough info for the outputFields to resolve with
'mutateAndGetPayload' => function( $input, $context, $info ) {
// Do any logic here to sanitize the input, check user capabilities, etc
$exampleOutput = null;
if ( ! empty( $input['exampleInput'] ) ) {
$exampleOutput = 'Your input was: ' . $input['exampleInput'];
}
return [
'exampleOutput' => $exampleOutput,
];
}
] );
Registering the above mutation would allow for the following mutation to be executed:
mutation {
exampleMutation(
input: { clientMutationId: "example", exampleInput: "Test..." }
) {
clientMutationId
exampleOutput
}
}
And the following response would be provided:
{
"data": {
"exampleMutation": {
"clientMutationId": "example",
"exampleOutput": "Your input was: Test..."
}
}
}
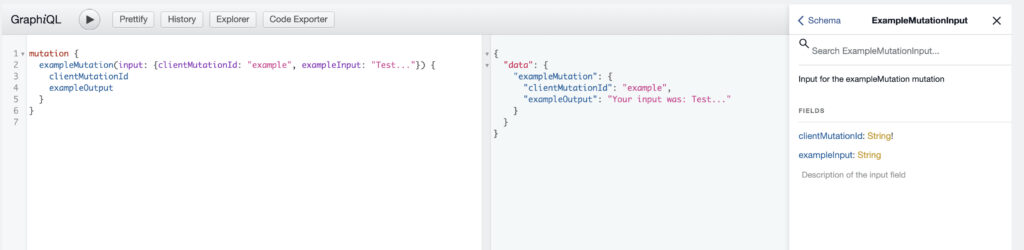