register_graphql_interfaces_to_types
Given a type name and interface name, this applies the interface to the Type.
register_graphql_interfaces_to_types( array $interface_names, array $type_names );
Parameters
- $interface_names (array): An array of interface names to register the types to
- $type_names (array): An array of type names to register the interfaces to
Source
File: access-functions.php
Examples
First an interface needs to be registered. This example adds an Interface Type called ExampleInterface
to the GraphQL Schema with a test field called exampleField
.
register_graphql_interface_type( 'ExampleInterface', [
'fields' => [
'exampleField' => [
'type' => 'String',
'resolve' => function() {
return 'This is an example';
},
],
],
]);
Now the ExampleInterface
can be registered to a GraphQL Type. In this example, it is registered to the User
object.
register_graphql_interfaces_to_types( ['ExampleInterface'], ['User'] );
You can now write a query:
{
users {
nodes {
exampleField
}
}
}
Which will return results similar to the following:
{
"data": {
"users": {
"nodes": [
{
"exampleField": "This is an example"
}
]
}
}
}
Here is how this looks inside GraphiQL:
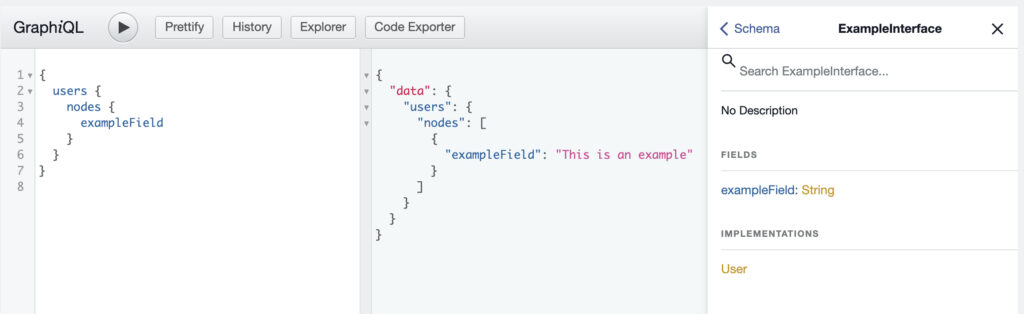