graphql_connection_page_info
Filter the pageInfo that is returned to the connection.
This filter allows for additional fields to be filtered into the pageInfo of a connection, such as “totalCount”, etc, because the filter has enough context of the query, args, request, etc to be able to calcuate and return that information.
apply_filters( 'graphql_connection_page_info', array $page_info, AbstractConnectionResolver $resolver );
Params
- $page_info (array): Array containing page info
- $resolver (AbstractConnectionResolver): Instance of the connection resolver
Example
You would want to register a “total” field to the PageInfo type, like so:
add_action( 'graphql_register_types', function() {
register_graphql_field( 'PageInfo', 'totalCount', [
'type' => 'Int',
'description' => __( 'The total count of items in the connection.', 'your-textdomain' ),
'resolve' => static function( $source, $args, $context, $info ) {
return $source['totalCount'];
},
] );
} );
Then, you would want to filter the connection query args to tell the underlying WP_Query to ask to count the total posts.
add_filter( 'graphql_post_object_connection_query_args', function( array $query_args, $source, $args, $context, $info ) {
$field_selection = $info->getFieldSelection( 2 );
if ( ! isset( $field_selection['pageInfo']['totalCount'] ) ) {
return $query_args;
}
$query_args['no_found_rows'] = false;
return $query_args;
}, 10, 5 );
You would also need to do the same for the other WordPress query classes. WP_Query uses no_found_rows => false
to tell SQL to count the total, but WP_Term_Query and
Then filter the pageInfo to return the total for the query, something to this tune:
add_filter( 'graphql_connection_page_info', function( $page_info, $resolver ) {
$page_info['totalCount'] = $resolver->get_query()->found_posts ?? null;
return $page_info;
}, 10, 2 );
This uses the results of the query to return the totalCount. Again, this would need to be more advanced to account for queries that return the data differently. WP_Query returns the data as found_posts
where other query classes might return differently.
Now, we can query connections like so, getting the total count:
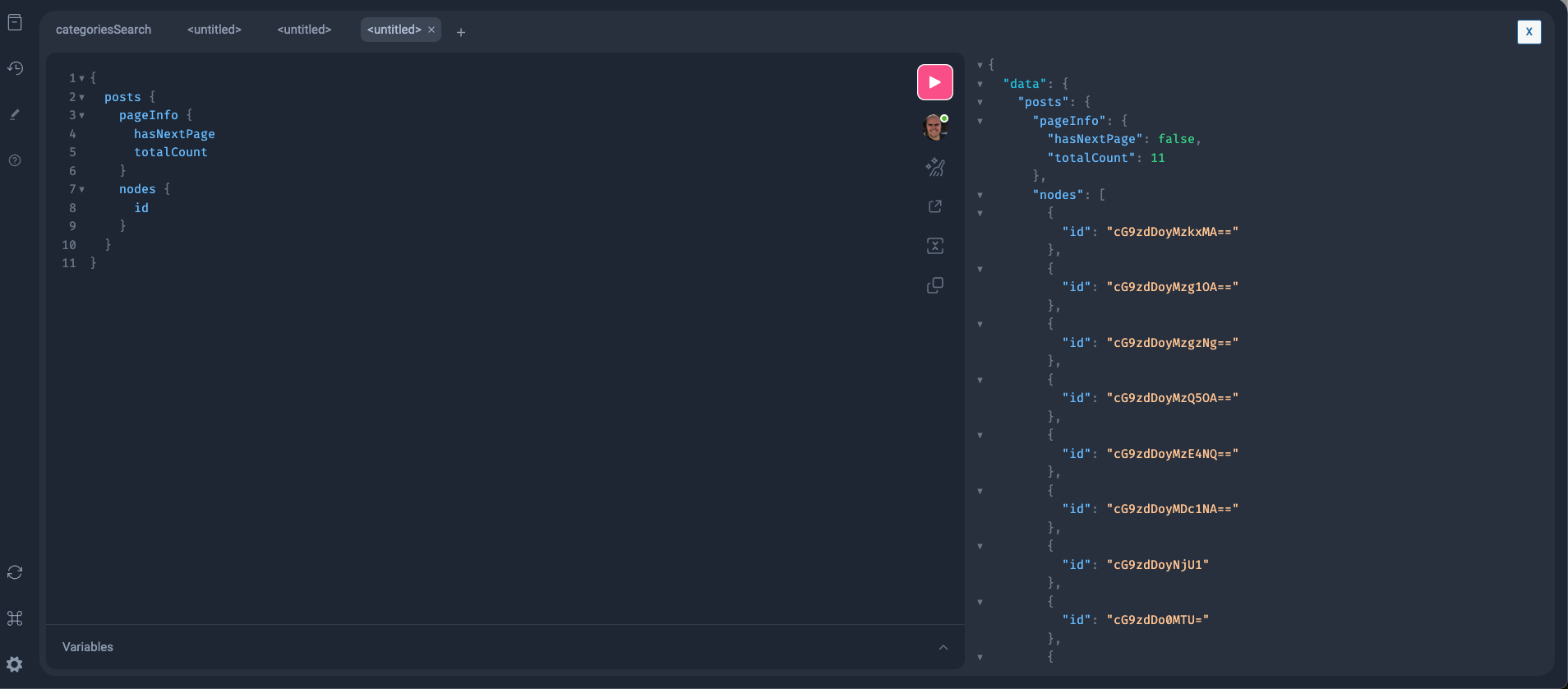